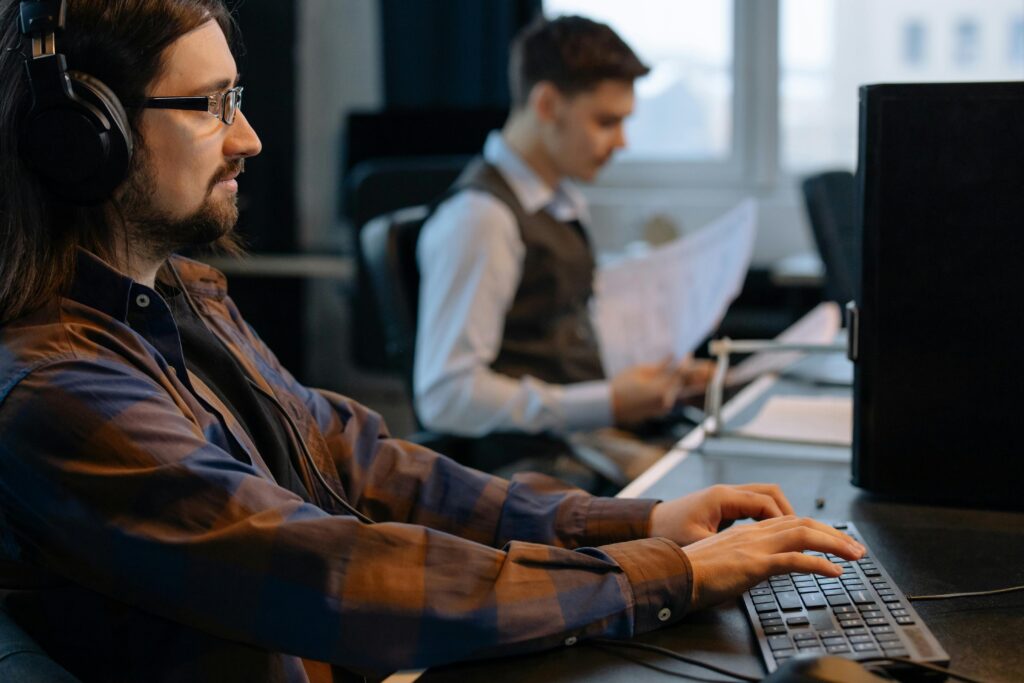
Getting Started with Flutter: A Comprehensive Beginner’s Guide
Introduction
Flutter is a powerful open-source UI toolkit developed by Google for building natively compiled applications for mobile, web, and desktop from a single codebase. If you’re new to Flutter, this tutorial will guide you through the basics and help you get started with creating your first Flutter app.
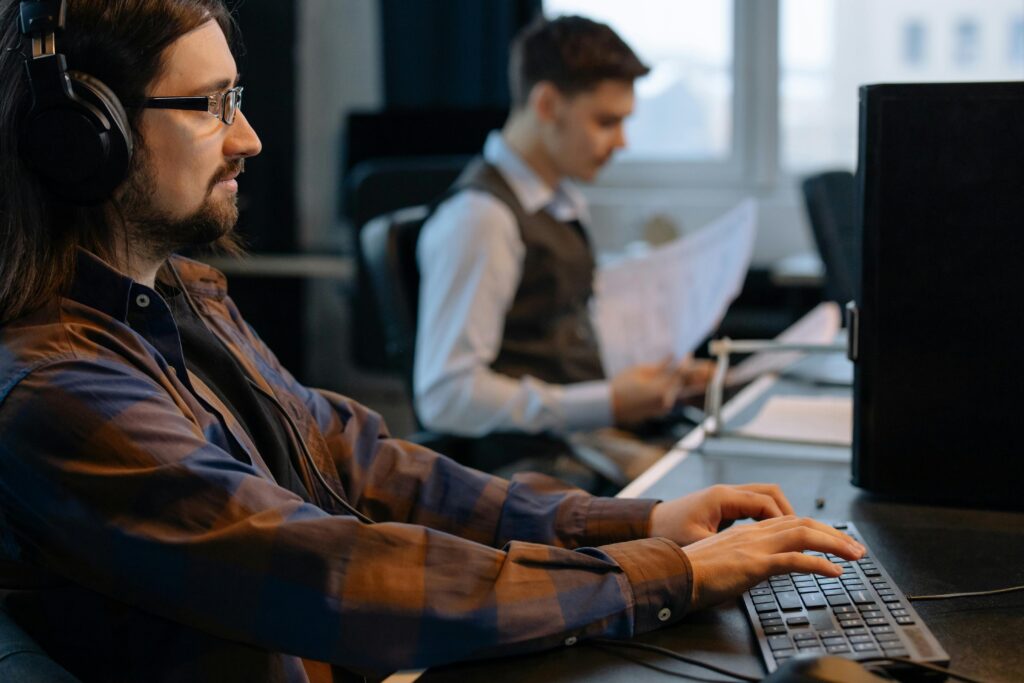
1. Introduction to Flutter
Flutter enables developers to build beautiful, fast, and native-quality applications for iOS, Android, and the web from a single codebase. It uses the Dart programming language and offers a rich set of customizable widgets and tools to streamline app development.
2. Setting Up Your Development Environment
Before you start coding with Flutter, you need to set up your development environment:
- Install Flutter SDK: Download and install the Flutter SDK from the official Flutter website (flutter.dev). Follow the installation instructions based on your operating system (Windows, macOS, Linux).
- Install Dart SDK: Flutter uses Dart as its programming language. Dart SDK comes bundled with Flutter, so no separate installation is required.
- Set Up an Editor: Use a code editor of your choice such as Visual Studio Code, Android Studio, or IntelliJ IDEA. Install the Flutter and Dart plugins/extensions for your editor.
3. Creating Your First Flutter App
Once your environment is set up, you can create a new Flutter project:
- Open your terminal or command prompt.
- Run the following command to create a new Flutter project:
flutter create myapp
Replace myapp
with the name of your application.
4. Understanding Flutter Widgets
Flutter revolves around widgets, which are building blocks of the user interface. Widgets can be structural (like rows, columns) or stylistic (like buttons, text). Flutter provides a rich set of pre-designed widgets and allows you to create custom widgets for your specific needs.
5. Building UI with Flutter
Flutter uses a declarative approach to build UIs, meaning you describe the desired UI state and Flutter handles all the UI updates. Here’s a basic example of a Flutter widget:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Tutorial'),
),
body: Center(
child: Text('Hello, Flutter!'),
),
),
);
}
}
6. Handling User Input and Navigation
Flutter provides widgets for handling user input (e.g., buttons, text fields) and navigation between screens. You can use stateful widgets to maintain UI state and update the UI in response to user interactions.
7. Working with Packages and Plugins
Flutter has a rich ecosystem of packages and plugins to extend its functionality. You can integrate third-party libraries for tasks like networking, database operations, authentication, and more. Use pub.dev
, the official Flutter package repository, to find and install packages for your project.
8. Testing and Debugging
Flutter offers tools like Flutter Inspector and Dart DevTools for debugging UI layouts, performance profiling, and analyzing app behavior. Write unit tests and integration tests using Flutter’s testing framework to ensure your app works correctly across different scenarios.
9. Deploying Your Flutter App
To deploy your Flutter app:
- Generate APK (Android) or IPA (iOS) files for distribution.
- Publish your app to Google Play Store or Apple App Store.
- Deploy web applications using Flutter for web.
Conclusion
Flutter empowers developers to create high-performance, visually appealing applications across multiple platforms with ease. This tutorial has provided you with the foundational knowledge to start building Flutter apps, from setting up your environment to creating UIs, handling user input, working with packages, testing, debugging, and deploying your applications. Explore Flutter’s extensive documentation, community resources, and sample projects to further enhance your Flutter development skills. Happy coding!