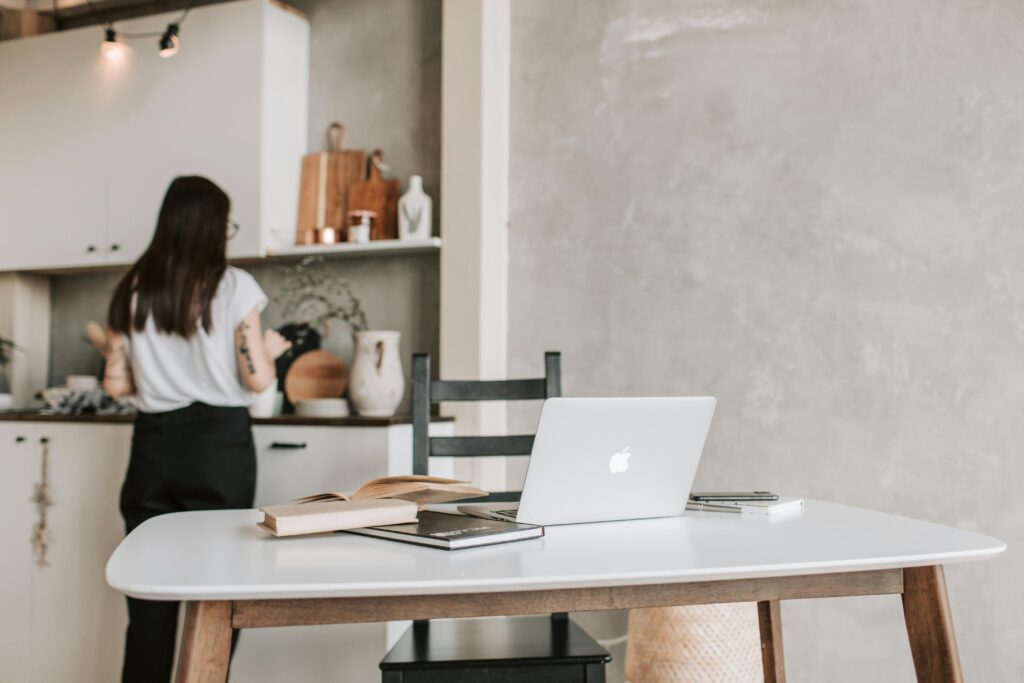
Getting Started with Ruby on Rails: A Beginner’s Guide
Introduction
Ruby on Rails, often simply called Rails, is a popular web application framework written in the Ruby programming language. It’s renowned for its simplicity, productivity, and convention-over-configuration approach. If you’re new to Ruby on Rails, this tutorial will introduce you to the basics and help you get started with building web applications.
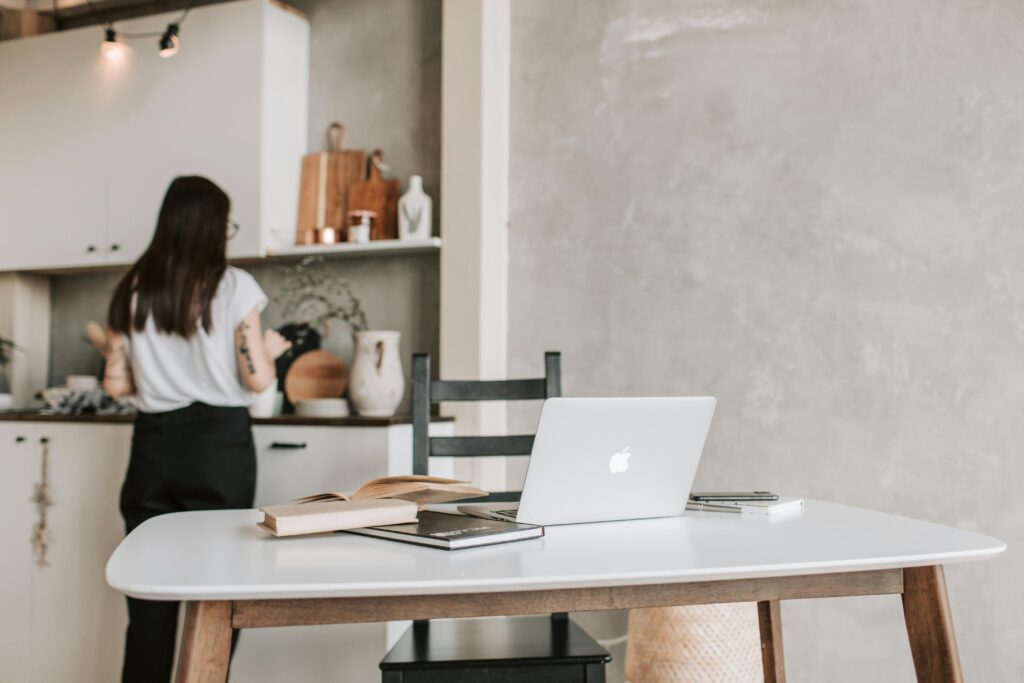
1. Introduction to Ruby on Rails
Ruby on Rails (Rails) is an open-source framework that follows the Model-View-Controller (MVC) architectural pattern. It emphasizes developer happiness and convention over configuration, allowing developers to focus on writing business logic rather than boilerplate code.
2. Setting Up Your Development Environment
Before you can start coding with Ruby on Rails, you need to set up your development environment. Here are the steps:
- Install Ruby: Rails requires Ruby to be installed on your system. You can install it using tools like RVM (Ruby Version Manager) or rbenv.
- Install Rails: Once Ruby is installed, you can install Rails using the gem package manager:
gem install rails
- Set Up a Database: Rails typically uses SQLite for development by default. You might also configure it to work with other databases like MySQL or PostgreSQL depending on your application’s needs.
3. Creating Your First Rails Application
Now that your environment is set up, let’s create a new Rails application:
- Open your terminal or command prompt.
- Navigate to the directory where you want to create your Rails application.
- Run the following command to create a new Rails app:
rails new myapp
Replace myapp
with the name of your application.
4. Understanding the MVC Architecture
Rails follows the MVC architectural pattern:
- Models: Handle data logic, such as database interactions and business rules.
- Views: Render HTML and present data to users.
- Controllers: Handle user requests, process input, and interact with models and views.
5. Generating Components
Rails provides generators to quickly create components like models, controllers, and views. For example:
- Generate a scaffold for a resource (e.g.,
Post
):
rails generate scaffold Post title:string content:text
This command generates a Post
model, a corresponding database migration, a controller with CRUD actions, and views for managing posts.
6. Routing and RESTful Design
Rails uses a routing system to map URLs to controllers and actions. Define routes in config/routes.rb
using a simple DSL (Domain-Specific Language) to create RESTful routes.
7. Adding Functionality
Once you have your basic application set up, you can start adding functionality:
- Define model associations (e.g.,
has_many
,belongs_to
). - Implement controller actions (e.g.,
create
,update
,destroy
). - Customize views using embedded Ruby (ERB) templates.
8. Testing Your Application
Rails comes with built-in support for testing. Use tools like MiniTest or RSpec to write and run tests to ensure your application behaves as expected.
9. Deploying Your Rails Application
When you’re ready to deploy your Rails application:
- Configure your production environment.
- Use deployment tools like Capistrano or Heroku.
- Monitor and maintain your application in production.
Conclusion
Ruby on Rails provides a powerful and efficient framework for building modern web applications. This tutorial has introduced you to the basics of Rails development, from setting up your environment to creating and deploying your first application. As you continue your journey with Rails, explore its vast ecosystem of gems, plugins, and community resources to enhance your development experience further. Happy coding!